In this tutorial, you will learn how to use RGB LED with Arduino. I am going to show how we can emit different colors using the RGB LED with Arduino.
Components Required
- 1 x Arduino UNO
- 1 x RGB LED
- 3 x 220 ohm Resistors
- Connecting Wires
- Breadboard
RGB LED
RGB stands for Red, Blue, Green. Unlike a normal LED which emit only one white color, RGB LED has three LEDs packed into one unit (LED). Red, Blue and Green colors are the primary colors, any color can be created upon mixing them but these colors cannot be created by mixing any of the colors.
It has four terminals
- Common Cathode
- Anode for Red
- Anode for Blue
- Anode for Green
In this tutorial, you will learn how we can connect and use an RGB LED with Arduino.
Circuit to Connect RGB LED with Arduino
The circuit is going to be very simple. We need an Arduino UNO Board, an RGB LED, 3 x 220 ohms resistors and a Breadboard.
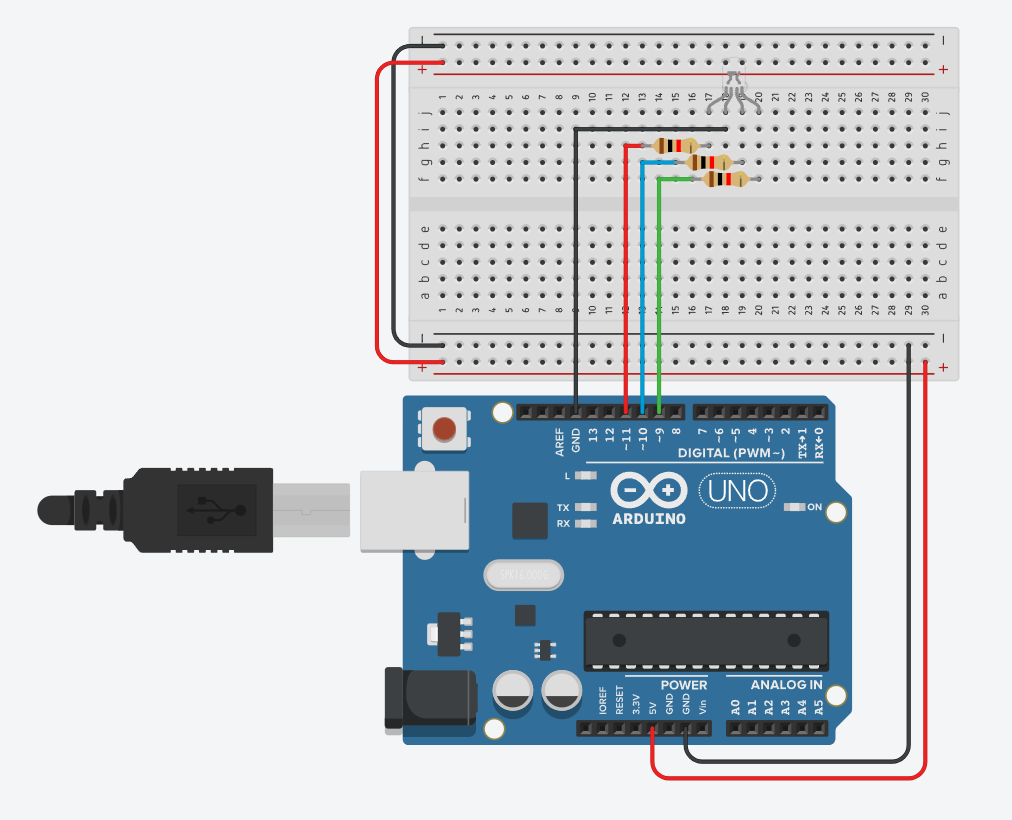
First of all connect the GND pin of Arduino UNO with the RGB LED’ Cathode. Here i am using PWM Pin 9, 10, and 11 with RGB LED’s Green, Blue and Red Pin respectively.
PINOUT
- Arduino PWM PIN 9 = RGB LED’s Green Anode
- Arduino PWM PIN 10 = RGB LED’s Blue Anode
- Arduino PWM PIN 11 = RGB LED’s Red Anode
- Arduino GND = RGB LED’s Cathode
Arduino Code
int redPin= 11;
int greenPin = 10;
int bluePin = 9;
void setup() {
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
}
void loop() {
setColor(255, 255, 255); // White Color
delay(1000);
setColor(255, 0, 0); // Red Color
delay(1000);
setColor(0, 255, 0); // Green Color
delay(1000);
setColor(0, 0, 255); // Blue Color
delay(1000);
setColor(245, 16, 16); // Orange Color
delay(1000);
}
void setColor(int redValue, int greenValue, int blueValue) {
analogWrite(redPin, redValue);
analogWrite(greenPin, greenValue);
analogWrite(bluePin, blueValue);
}
The Code is very simple. I declared three variables redPin, greenPin and bluePin and set these pins of arduino to OUTPUT using pinMode in void setup() .
In the void loop(), the function we are using is setColor(). Which takes three values first value is for Red color, second value is for Green Color and third value is for Blue Color. As we are using PWM pins of arduino which can take value from 0 to 255. So in order to emit Red color we need to set the value of Red to 255 while keeping the values of Green and Blue to 0. Similarly the other two primary colors can be emitted.
In order to emit white color we need to set the value of each primary color to at its maximum 255.